
Heaps are usually implemented as binary trees.
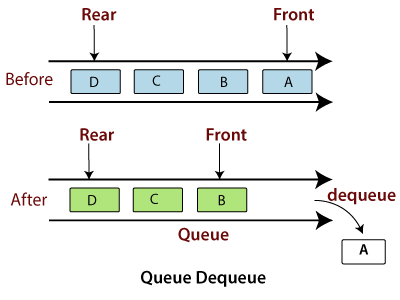
This allows trees to grow organically to multiple layers. The root can have child nodes, and each child node can also have children. Trees are hierarchical data structures containing a parent node, which is called the root. A heap is a special type of tree-based data structure.

Queues are efficient in Python because they are implemented as heaps. They are also the best choice for evaluating mathematical expressions, because of the importance of order of operations. In programming, most compilers extensively use stacks. When more stock is necessary, the top items are removed first. New supplies are placed on top of older orders. For instance, stacks are used to store and retrieve non-perishable supplies. Stacks are less obvious in day-to-day life, but are used whenever efficiency is preferred over strict fairness. The most recent item to arrive is always the next item to be selected. A stack is also a list-based data structure, but it uses a last in first out (LIFO) scheme. The next item to arrive would be added to the end of the queue, following E. Item D is at the front and would be the next scheduled item, followed by E. Items B and C are retrieved because they occupy the first two positions of the queue.
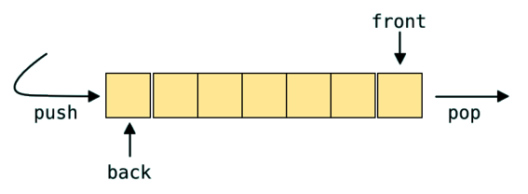
They make programs easier to understand and write, and often faster and more reliable too.Ĭonceptually, a queue represents data items as an ordered list. Data structures are used to organize, manage, and store data.

Queues in Python What is a Queue?Ī queue is a fundamental programming data structure.
#OBJECT COMPARISON IN PRIORITY QUEUE PYTHON HOW TO#
This guide introduces the Python priority queue and explains how to implement it in Python 3. Prioritization can be complicated, but fortunately Python priority queues can be easily and efficiently implemented using a built-in module. A queue that retrieves and removes items based on their priority as well as their arrival time is called a priority queue. However, it is often necessary to account for the priority of each item when determining processing order. In Python, queues are frequently used to process items using a first in first out (FIFO) strategy.
